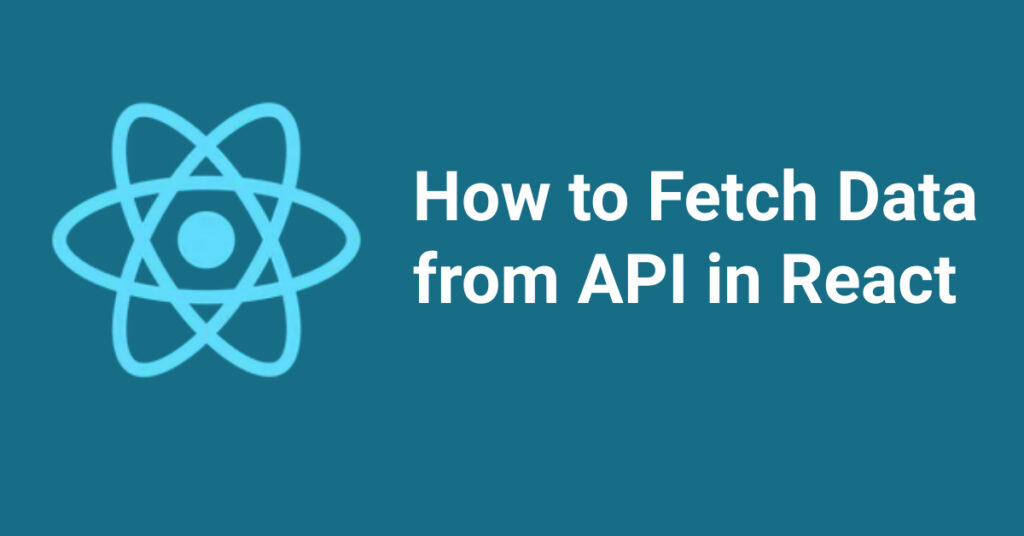
When designing applications, you frequently need to access data via an API. This allows you to present dynamic, frequently updated content within your application.
In this article, I’ll show you different methods of fetching data using React.
What is a API
API stands for Application Programming Interface. It allows information and functionality to be exchanged across several systems, such as a website and a server or many software programs.
How to Fetch Data in React
Following are the methods that we can use to fetch data from API
- Using Fetch
- By Axios
There are other methods by which you can fetch data from API but less popular
- By SWR Method
- By React Query
Now going to demonstrate how Fetch
works:
The fetch()
method is often used for retrieving data from APIs. It is regarded as the simplest and most often utilized method.
The advantages of using the fetch()
method
- The
fetch()
method makes it simple to get information from the internet using JavaScript. - It allows you to submit more information to the server, such as who you are and what type of data you want.
- It’s intended to perform well in the majority of modern web browsers.
- The
fetch()
method supports differentHTTP
methods. These methods include get, post, put, and delete. They give you flexibility in interacting with APIs. Fetch()
is a native JavaScript method. It doesn’t require any other libraries or dependencies. This makes it light and efficient.
How to use fetch()
to get data from API
- Create a file called
fetchUsers.js
- Then import
useState
to manage data from API - Finally import
useEffect
to control API call
Here’s fetchUsers.js
import React, { useState, useEffect } from 'react';
const FetchUsers = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/users')
.then((res) => {
return res.json();
})
.then((data) => {
setUsers(data);
});
}, []);
return (
<div>
{users.map((user) => (
<p>{user.name}</p>
))}
</div>
);
};
export default FetchUsers;
Within useEffect()
, we retrieve our data by making a request using the API key. The response is returned as JSON (JavaScript Object Notation).
In the return statement, we use a map()
function to traverse through the incoming photos.
Now import this component in App.js
Here’s how App.js
file look like
import React from "react";
import './App.css';
import FetchUsers from "./FetchUsers";
function App() {
return (
<div className="App">
<header className="App-header">
<FetchUsers />
</header>
</div>
);
}
export default App;
And once we reload the app then how it will look on browser
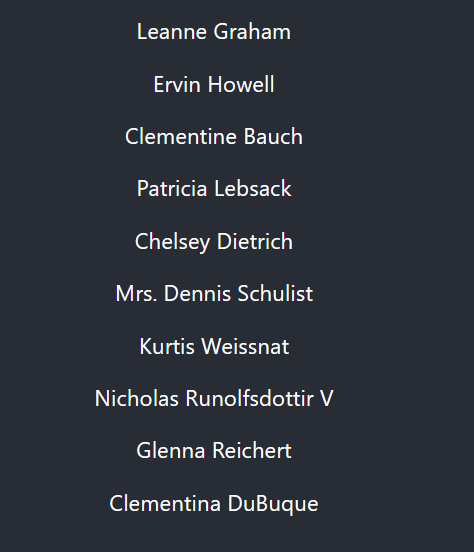
Conclusion, there are several methods to fetch data from API but we see that like how we can fetch data from Fetch in React. you can use other methods as well if you want but most popular is fetch and Axios
Great Article! Well Explained