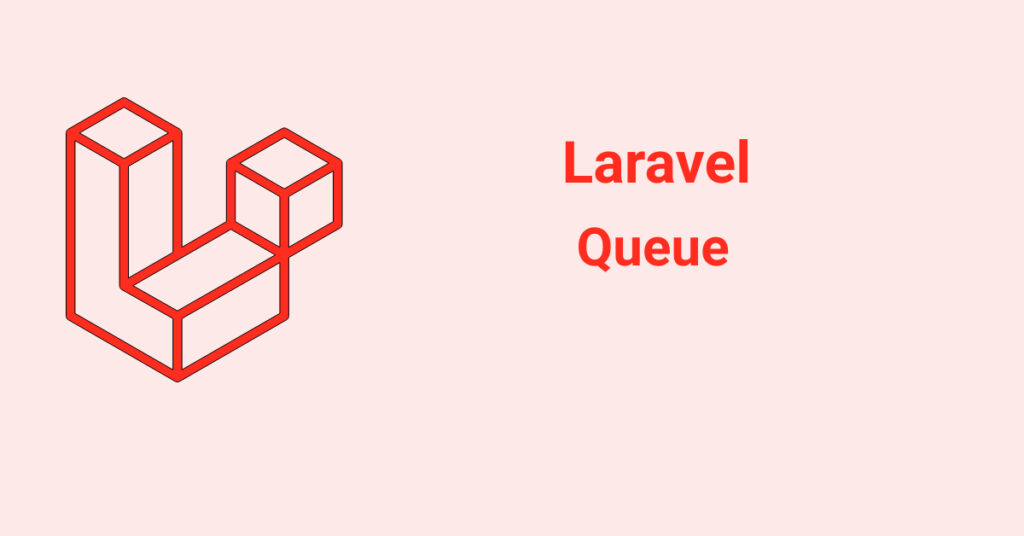
Laravel is a popular PHP online application framework that offers developers a wide range of features and capabilities, including an efficient job processing mechanism known as queues. Queues allow you to defer time-consuming processes, such as sending emails or generating reports, so that your application can handle other requests.
In this article, we’ll go over how to set up and use Laravel queues, as well as provide some examples to demonstrate their actual application.
Step 1: Configure your Queue Driver
Laravel supports a variety of queue drivers, including Redis, Amazon SQS, and Beanstalkd. You can choose the right driver for your application depending on its individual needs and environment.
Laravel provides other local driver called database, you can choose this driver if you don’t go third party drivers
To configure the queue driver, visit to the .env
file in your Laravel application root directory and update the QUEUE_CONNECTION
variable to the desired driver:
QUEUE_CONNECTION=redis
Step 2: Create a Job Class
Next, define a job class that represents the task you wish to complete.
Job classes should implement
the Illuminate\Contracts\Queue\ShouldQueue
interface to indicate that the job can be added to the queue.
Let’s create a job that sends an email to a user.
php artisan make:job WelcomeEmailJob
This creates a new job class in the app/Jobs directory. The handle method allows you to define the job’s logic:
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Facades\Mail;
class WelcomeEmailJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $user;
public function __construct($user)
{
$this->user = $user;
}
public function handle()
{
Mail::to($this->user->email)->send(new \App\Mail\WelcomeEmail($this->user));
}
}
Step 3: Dispatch the Job to the Queue
Once you’ve defined your job class, use the dispatch
method to send it to the queue. For example, let’s send the WelcomeEmailJob using the user object as the parameter:
$user = User::find(1);
dispatch(new WelcomeEmailJob($user));
Alternatively, you may use the dispatchNow
method to run the job instantly without adding it to the queue:
Step 4: Process the Queue
To begin processing the queued jobs, you must first launch a queue worker. Laravel includes many queue workers, including the php artisan queue:work
command, which launches a worker that continuously polls the queue and processes jobs as they become available.
php artisan queue:work
The --queue
and --tries
options additionally allow you to specify the queue name and the number of worker threads.
php artisan queue:work --queue=emails --tries=3
This will start a worker that processes jobs from the email
queue and retries failed ones up to three times.
Step 5: Monitor the Queue
The Laravel Horizon package provides a dashboard that displays information about queue workers, jobs, and failed jobs, allowing you to keep track of the status of your queued jobs.
To install and configure Laravel Horizon, run the following command:
composer require laravel/horizon
Finally, Laravel queues are an effective approach to manage time-consuming operations in the background, freeing up your application’s resources to handle additional requests. By following the instructions indicated in this article, you can quickly configure and use Laravel queues in your application. Laravel Horizon allows you to monitor and manage the queue, ensuring that your application runs smoothly and efficiently. Laravel queues allow you to create a better user experience for your customers while also improving the overall efficiency of your application.